How to call server side function from client side javascript in ASP.Net?
How to call serverside function from client side javascript in ASP.Net?
Call ASP.Net/C# function using Javscript
Usually in ASP.net platform, server side functions (all codes written in asp.net,C#) are executing from the server side and all client side funtions (javascript,jquery,ajax) are executing from the client side. Obviously client side functions are too faster than the server side because there is no need to go upto server, will running from the browser itself.
But we are unable to written all functions in client side to get good performance. But for this technique, there is some tips can be used. What we are going to do is, we are writing a server side code in ASP.Net,C# and call these functions from client side using javascript and ajax.
Steps to Calling ServerSide method from javscript
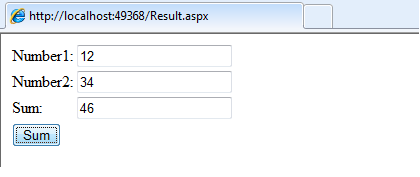
- Create a aspx page with three textboxes and a button control.
- User enter two numbers in first two textboxes and press calculate button.
- We are fetching entered values and calling a serverside funtion by passing this values.
- In the server side function find the sum and return the result.
- In client side’s succes event, getting result from the server and displayed to third textbox.
Step 1 :
Create a aspx page with controls, two textboxes for entering the numbers, one button for find the sum of number and a one more text box to display the result.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Result.aspx.cs" Inherits="testWebApp.Result" %> <%@ Register Assembly="AjaxControlToolkit" Namespace="AjaxControlToolkit" TagPrefix="ajaxToolkit" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <asp:ScriptManager ID="sm" runat="server" EnablePageMethods="true"> </asp:ScriptManager> <div> <table> <tr> <td> Number1: </td> <td> <asp:TextBox ID="txt1" runat="server"></asp:TextBox> </td> </tr> <tr> <td> Number2: </td> <td> <asp:TextBox ID="txt2" runat="server"></asp:TextBox> </td> </tr> <tr> <td> Sum: </td> <td> <asp:TextBox ID="txtSum" runat="server"></asp:TextBox> </td> </tr> <tr> <td> <asp:Button ID="btnSum" runat="server" Text="Sum" OnClientClick="CallSum();return false;" /> </td> </tr> </table> </div> </form> </body> </html>
Step 2
In code behind, write a web method to access two parameters and return the result. In the function we added parameters and return the result.
protected void Page_Load(object sender, EventArgs e) { } /// <summary> /// Server side function Sum /// </summary> /// <param name="arg1">arg1</param> /// <param name="arg2">arg2</param> /// <returns>result(sum)</returns> [System.Web.Services.WebMethod] public static int Sum(int arg1, int arg2) { /* On server side we can do any thing. Like we can access the Session. * We can do database access operation. Without postback. */ try { return arg1 + arg2; } catch (Exception ex) { throw ex; } }
Step 3
Create a javscript method in aspx page to get input textboxes values and call serverside function. Here itself we decalre a success and failure event, for getting the event from the server after the current function executed.
<script language="javascript" type="text/javascript"> <!-- // Javascript function function CallSum() { //Get the controls var txt1 = $get("txt1"); var txt2 = $get("txt2"); var txtresult = $get("txtSum"); //Call server side function PageMethods.Sum(txt1.value, txt2.value, OnCallSumComplete, OnCallSumError, txtresult); /*Server side function get the 2 arguments arg1 and arg2. We are passing txt1.value and txt2.value for that. OnCallSumComplete is callback function for complete successfully. OnCallSumError is callback function on error. txtresult is usercontext parameter. OnCallSumComplete,OnCallSumError,txtresult are optional parameters. If server side code executed successfully then OnCallSumComplete will call. If server side code do not executed successfully then OnCallSumError will call. */ } // Callback function on complete // First argument is always "result" if server side code returns void then this value will be null // Second argument is usercontext control pass at the time of call // Third argument is methodName (server side function name) In this example the methodName will be "Sum" function OnCallSumComplete(result, txtresult, methodName) { //Show the result in txtresult txtresult.value = result; } // Callback function on error // Callback function on complete // First argument is always "error" if server side code throws any exception // Second argument is usercontext control pass at the time of call // Third argument is methodName (server side function name) In this example the methodName will be "Sum" function OnCallSumError(error, userContext, methodName) { if (error !== null) { alert(error.get_message()); } } // --> </script>
Project is ready for call server sided functions from client side using javascript. Run the application and enter two numbers in first and second textboxes then press calculate button, the we can show that result is displayed in third box without any page postback. If we enterd alphabets instead of numbers, from the server it will happening error and ‘failure’ event occurred in the javascript will alert the details of the error.
Reference :
Comments